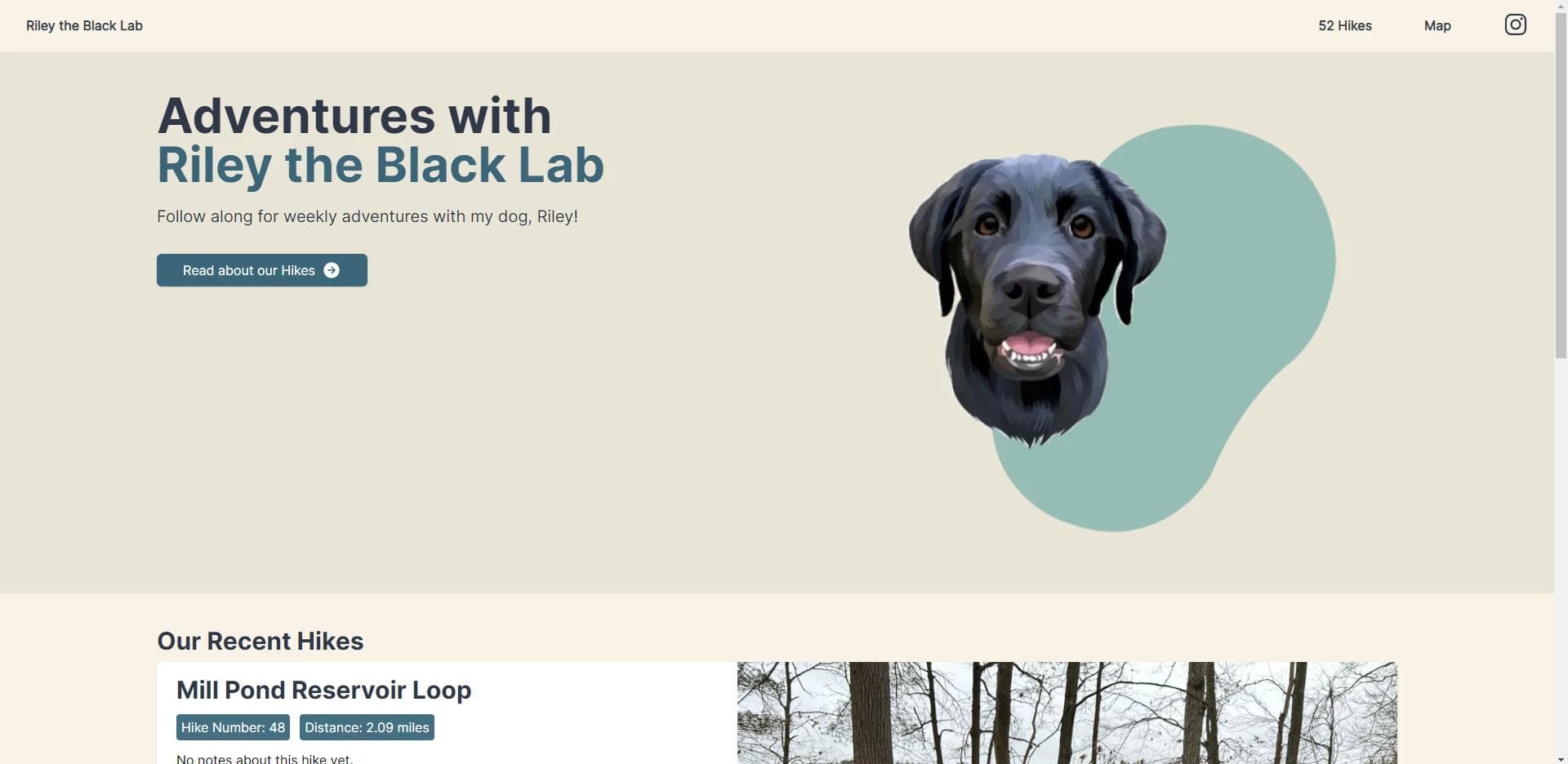
Black Lab Riley
January 22, 2023
This was a personal project I used to help me learn the ins and outs of NextJS making use of Sanity as a headless CMS
NextJS
Sanity.io
Building a Blog with NextJS
I wanted to learn how to build a dynamic blog website that could generate as many pages as I needed depending on the amount of blogs. At the same time of wanting to learn NextJS and Sanity.io, I was also starting a hiking challenge with my dog, Riley. I figured this would be the best way to practice my development skills.
Headless CMS
A headless CMS is a content management system that is able to be use with many different tech stacks. It specifically makes use of an API (application program interface) which fetches data and then gives the website a response with that data. I choose Sanity.io for content management. Sanity has plenty of documentation and an abundance of tutorials on how to integrate it with NextJS.
Writing my first schema
export default {
name: 'post',
title: 'Post',
type: 'document',
fields: [
{
name: 'title',
title: 'Title',
type: 'date',
},
{
name: 'slug',
title: 'Slug',
type: 'slug',
options: {
source: 'title',
maxLength: 96,
},
},
{
name: 'publishedAt',
title: 'Published at',
type: 'datetime',
},
{
name: 'mainImage',
title: 'Main image',
type: 'image',
options: {
hotspot: true,
},
fields: [
{
name: 'alt',
title: 'Alternative Text',
type: 'string'
}
]
},
{
name: 'body',
title: 'Body',
type: 'blockContent',
},
],
preview: {
select: {
title: 'title',
media: 'mainImage',
},
prepare(selection) {
const {author} = selection
return Object.assign({}, selection, {
subtitle: author && `by ${author}`,
})
},
},
}
This was my first time really working with schemas and I quickly began to understand how the database was being built based on fields that I set and the returning JSON (JavaScript Object Notation). This was exciting for me as I've seen JSON used at my job but didn't know how to use or build it.
Because NextJS complies JavaScript code into HTML upon user or server request, this allowed me to use variables that were fetched from my database in the page such as the title or bodyContent.
Google Map API
Another big feature of this website is the use of the Google Map API, this allows developers to place components on a Google Map iframe based on geographical coordinates. I figured this was a great project to practice this on because I was hiking all over New England and having a digital map of all the places I hiked would be really cool.
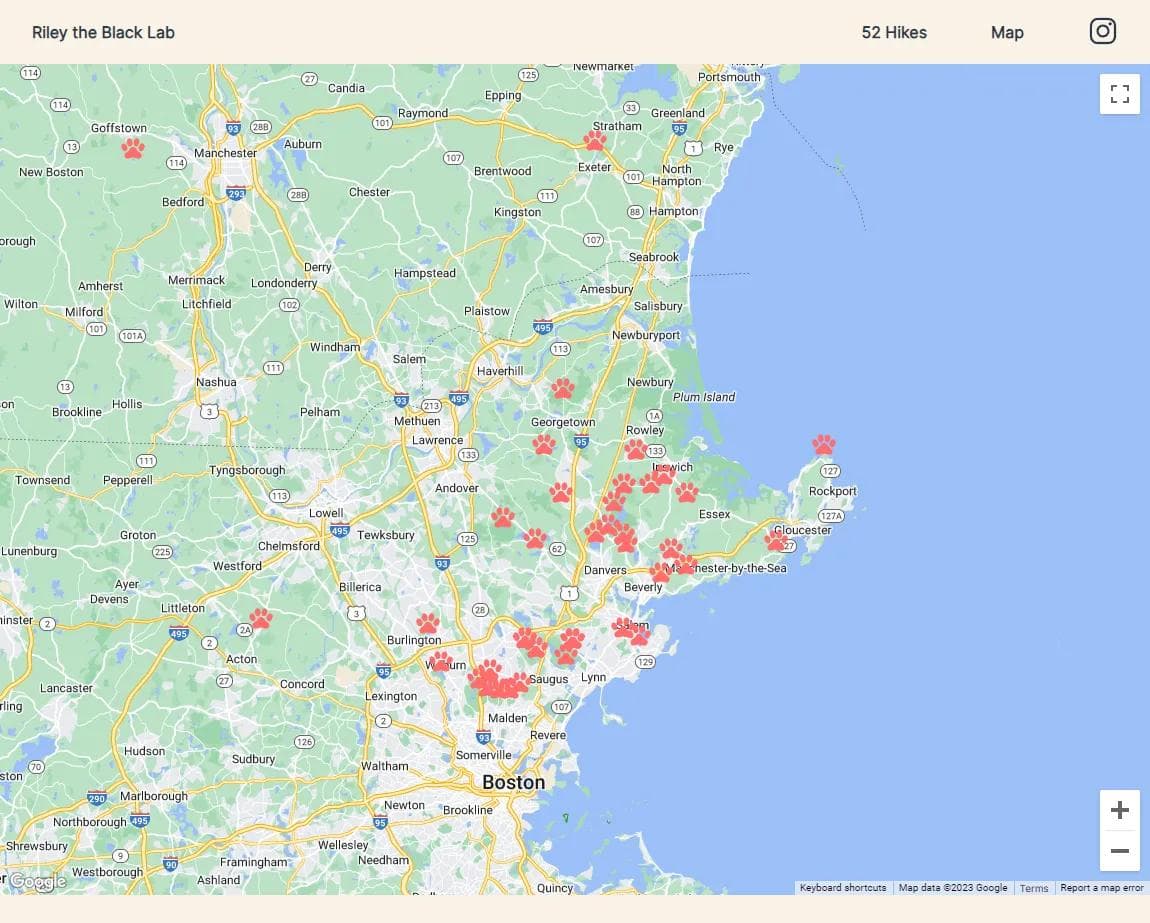